13 minutes
Tekton Golang pipeline with Signed Provenance - SLSA Level 2
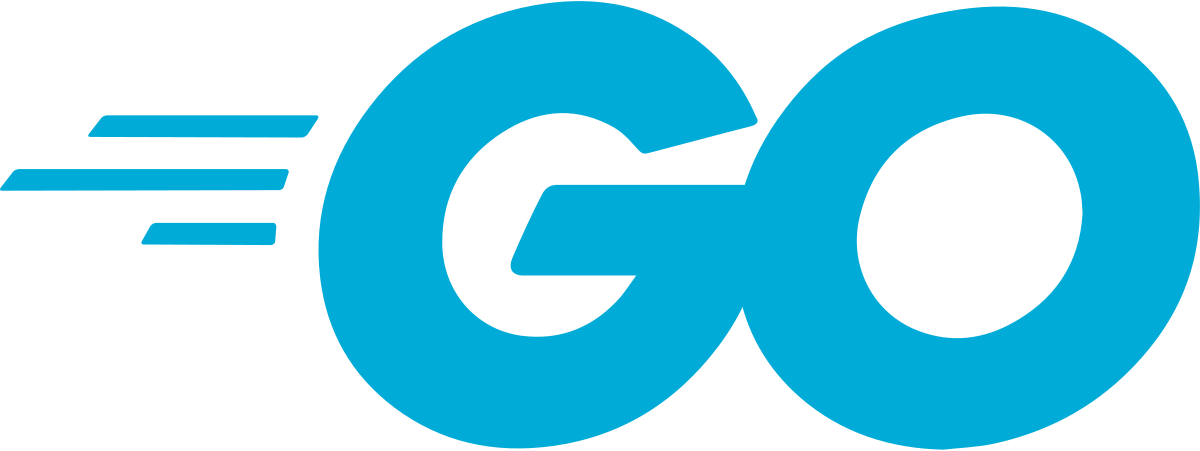
Tekton Pipelines and Tekton Chains provide many benefits when creating cloud native CD/CD systems. In my previous secure supply chain post, I spoke about what are the different components of Tekton and how we can combine pipelines and chains to create a SLSA level 2 compliant CI/CD pipeline. Today we will dive in deeper and create a more complex GO pipeline that will help us clone, build, run unit tests, create the image and scan the image. Tekton Chains will run along side pipelines in the end to provide us with a signed provenance that will be stored with the image in an OCI registry!
Overview of Tekton Pipelines and Chains
Be sure to check out my previous secure supply chain webinar to get some details on Tekton and all the tools that it provides. Lets have a quick recap of Tekton Pipelines and Chains before we get started.
Tekton Pipelines is an open-source framework for creating Cloud Native CI/CD systems, allowing developers to build, test, and deploy across cloud providers and on-premise systems.
Tekton Chains is a Kubernetes Custom Resource Definition (CRD) controller that allows you to manage your supply chain security in Tekton. It works together with Tekton Pipelines and provides the following captabilities (more to be added in the future)
- Signing TaskRun results with user provided cryptographic keys, including TaskRuns themselves and OCI Images
- Attestation formats like intoto (SLSA Provenance)
- Signing with a variety of cryptograhic key types and services (x509, KMS)
- Support for multiple storage backends for signatures
Deploy and Configure Tekton
Currently the SPIRE agent is deployed as a DaemonSets
where each node has workload API exposed. Each workload would mount the workload API as a hostPath
volume. The motivation for the CSI driver creation was to remove the need for the workload pods to mount the workload API. Thus, only the SPIRE agent pod that contains the CSI driver containers would require the hostPath
volume mounts (to interact with the Kubelet). This is the only limitation of this driver as using an emptyDir
volume would result in the backing directory to be removed if the SPIFFE CSI Driver pod is restarted, invalidating the mount into workload containers.
Pre-requisite:
- A running Kubernetes cluster (Docker Desktop Kubernetes, k3d, minikube)
- Cosign Installed (this will be used for sigining the image)
- If you have Go 1.16+, you can directly install by running:
- If you are using Homebrew (or Linuxbrew), you can install cosign by running:
Deploying Tekton:
Note: You can find a script and configs of this example on my github: tekton-golang-pipeline
First we will install and run Tekton Pipeline and Tekton Chains locally in our cluster before we can create our pipeline. This can be done by:
- Run the following command to install Tekton Pipelines and its dependencies:
- Monitor the installation using the following command until all components show a Running status:
- To install the latest version of Chains to your Kubernetes cluster, run:
- To verify that installation was successful, wait until all Pods have Status Running:
Note: Tekton Chains current requires the following results to be output from a task that creates an OCI Image: *IMAGE_URL - The URL to the built OCI image *IMAGE_DIGEST - The Digest of the built OCI image
where * indicates any expression. For example, if both MYIMAGE_IMAGE_URL AND MYIMAGE_IMAGE_DIGEST are correctly formatted to point to an OCI image, then chains will pick up on it and try to sign the image.
Configuring Chains
Tekton Chains requires a key pair that will be used for signing. We will use cosign to create this private/public key pair for us and store it as a secret in the tekton-chains
namespace called sigining-secrets
. Tekton Chains will automatically pick up this secret and sign the taskruns and images with it.
Next we will need to change the default configuration of Tekton Chains to use the in-toto format (which is the current SLSA format) and store the payload and signature into an OCI registry. This can be modified using the below commands.
Add in Tekton Tasks
We will be utilizing some of the pre-defied tasks in the Tekton Catalog. This is a great starting place for new users to start using tasks, modifying existing to fit their needs or creating their own from pipelines. Specifically we will be using the following task to create out pipeline:
- git-clone
- Clone down the repo to build
-
- golang-test
- Run unit tests on the golang project before building
-
- golang-build
- Build the golang project
-
- trivy-scanner
- Trivy (tri pronounced like trigger, vy pronounced like envy) is a simple and comprehensive scanner for vulnerabilities in container images, file systems, and Git repositories, as well as for configuration issues.
-
- kankio
- Kaniko is used to build container images from a Dockerfile, inside a container or Kubernetes cluster. This is chains compliant and contains the *IMAGE_URL and *IMAGE_DIGEST in the results.
-
Creating our Golang Pipeline
Now that all the Tekton tasks are loading into our kubernetes cluster, we can create the pipelie and pipelinerun to stage and execute the task with specific parameters.
First we need need a pesistent volume claim, that will be used as a shared workspace for the tasks as they run in the pipeline:
Next it will be the pipeline itself. This will take the tasks we loaded before and create a pipeline. In the tasks
section below you can see we reference: git-clone, golang-test, golang-build, kaniko and trivy-scanner (once for filesystem scan and the other image scan). The PVC (persistent volume claim) is used as a workspaces
that is mounted with the tasks as a shared work space. The params
section will be used to pass in the variables into the specific tasks. This allows for the pipeline (and subsequently the tasks) to be reusable.
Testing our Golang Pipeline
Now that all the pieces (task, pipeline, pipelinerun) are in place, we can test out our pipeline and see what it outputs.
Visualization
To view the results of the pipelinerun and taskruns, you can use the usual kubectl
commands and interact with the CRDs (kubectl get taskruns
or kubectl get pipelinerun
). Tekton provides two other methods to view the results: Tekton Dashboard and Tekton CLI. This are both optional and not needed for Tekton Pipeline and Chains to work.
Tekton Dashboard
You can install Tekton Dashboard by:
Once the dashboard is running you can run the following command to port-forward the dashboard. This will allow you to view it in your web browser:
Browse http://localhost:9097 to access your Dashboard.
Tekton CLI
To install the Tekton CLI, there are multiple methods based on your OS. Navigate to the Tekton CLI repo to install.
Running the demo
In order to instantiate the pipeline and the tasks within it we need to create the pipelinerun. We pass in the specific params
that we will be using for the test run. pipelineRef
references the pipeline-go-test
we created above. Workspaces
maps to the PVC we created pipelinerun-go-test-source-ws-pvc
.
Note: The below PipelineRun is missing the <IMAGE_NAME>
. Be sure to replace this with a repository that you want kaniko to push the image to. This can be docker hub or some other private or public repo that you have access to. In the example, I am using ttl.sh (anonymous & ephemeral Docker image registry). Specifically I used ttl.sh/example-123/go-build-test
but you can anything name for your repo example-123
and image name go-build-test
.
Save the file accordingly and run kubectl create -f
for the pipelinerun as it gernerateName
a name when its instantiated.
If you initialized the tekton dashboard you can view the pipelinerun from your browser:
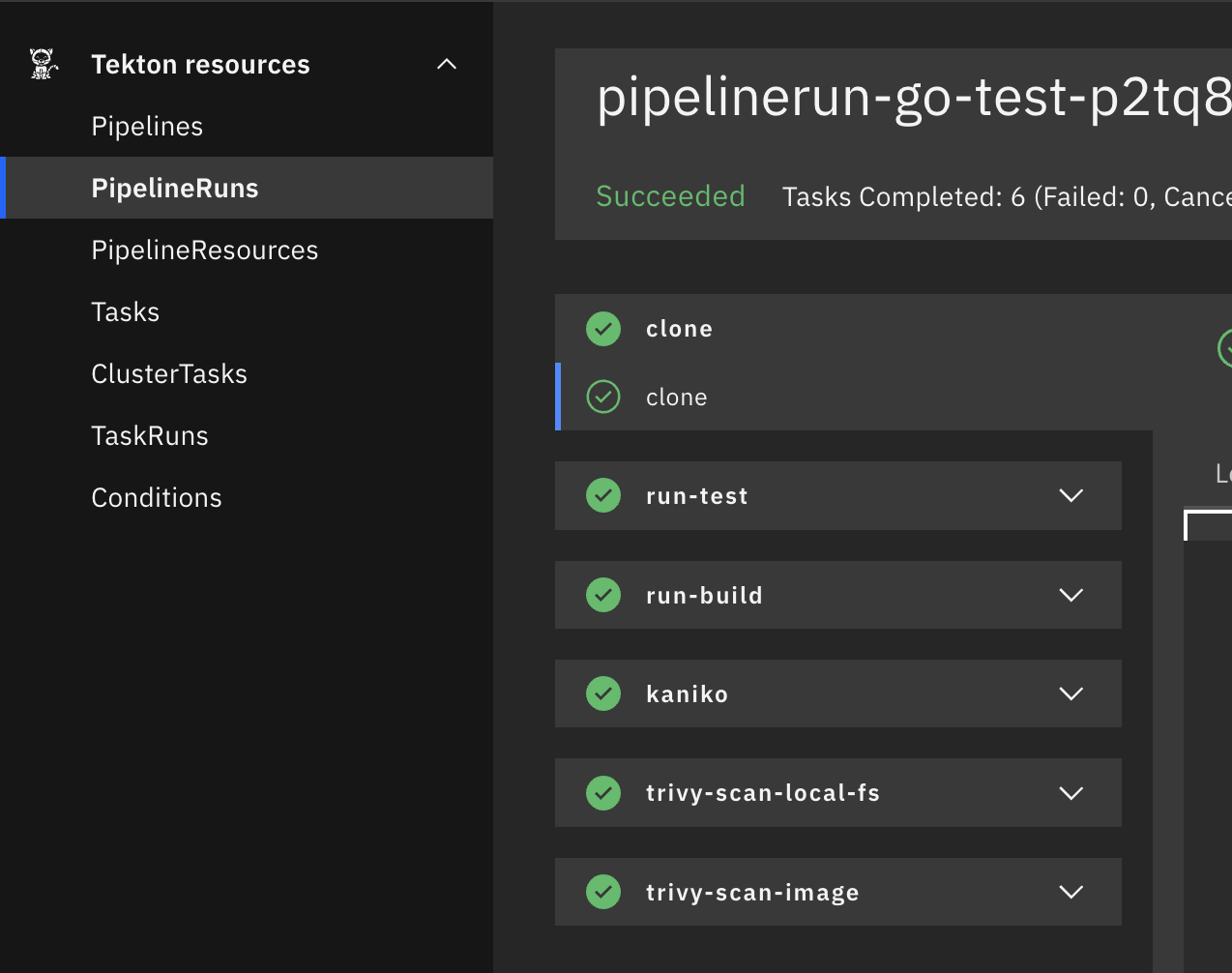
You can also view via Tekton CLI using the following command tkn pr describe
:
Note: If you are having trouble getting things to run, you can visit our github to find the full example and script to get it running quickly: tekton-golang-pipeline
Conclusion
Viewing the Results:
Once the pipeline finishes, you can view the logs from each task. For example, you can view the unit tests that have been run either via the tekton dashboard or tekton CLI.
Note: Replace with your pipelinerun name
We can also view the trivy image scans that ran after the image was created:
Note: Replace with your pipelinerun name
Verifying the Image and Attestation:
We can use cosign to verify the image and the attestation created by Tekton Chains are signed and stored in OCI registry.
Replace <IMAGE_NAME>
with what was used when running the pipelinerun above.
The output from the verify
will be similar to the below. This shows us that the image is indeed signed by our private key we created at the start with cosign. This also allows us to trust that the image was created by our pipeline.:
Next we will verify the attestation being created and stored in OCI by Tekton Chains.
Replace <IMAGE_NAME>
with what was used when running the pipelinerun above.
The output from the verify-attestation
will be similar to the below. The attestation is once again signed by our private key. The payload is base64 encoded and can be decoded to view the attestation for the image created by the pipeline.:
All done and verified! All the configs and examples for this blog are located on my github page: tekton-golang-pipeline
Stay tuned for more posts in the future for more tools that combine both automation and security!